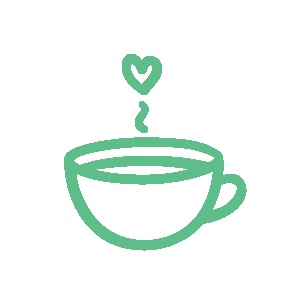
Producer consumer example
There might be conflict on buffer[], but consumed_until and produced_until prevent that (if used correctly).
So, only need to make sure consumed_until and produced_until accessed correctly under concurrency.
Using locks
In each round of produce()
1 | while (true) { |
Two locks:
- check if can produce: conflict on consumed_until
- conflict on produced_until
In each round of consume()
1 | while (true) { |
Two locks:
- check if can consume: conflict on produced_until
- conflict on consumed_until
Using atomic vairables
In produce, two locks can be modified:
check if can produce: conflict on consumed_until
no ordering constraints -> memory_order_relaxed
conflict on produced_until
store produced_until should happen after all operations -> load-store + store-store = release
1 | while (true) { |
In consume, two locks can be modified:
check if can consume: conflict on produced_until
load produced_until should happen before all operations -> load-load + load-store = acquire
conflict on consumed_until
store consumed_until should happen after all operations -> load-store + store-store = release
1 | while (true) { |
Memory barriers
memory_order_relaxed: no ordering constraints
memory_order_consume
memory_order_acquire: load-load + load-store
memory_order_release: load-store + store-store
memory_order_acq_rel
memory_order_seq_cst
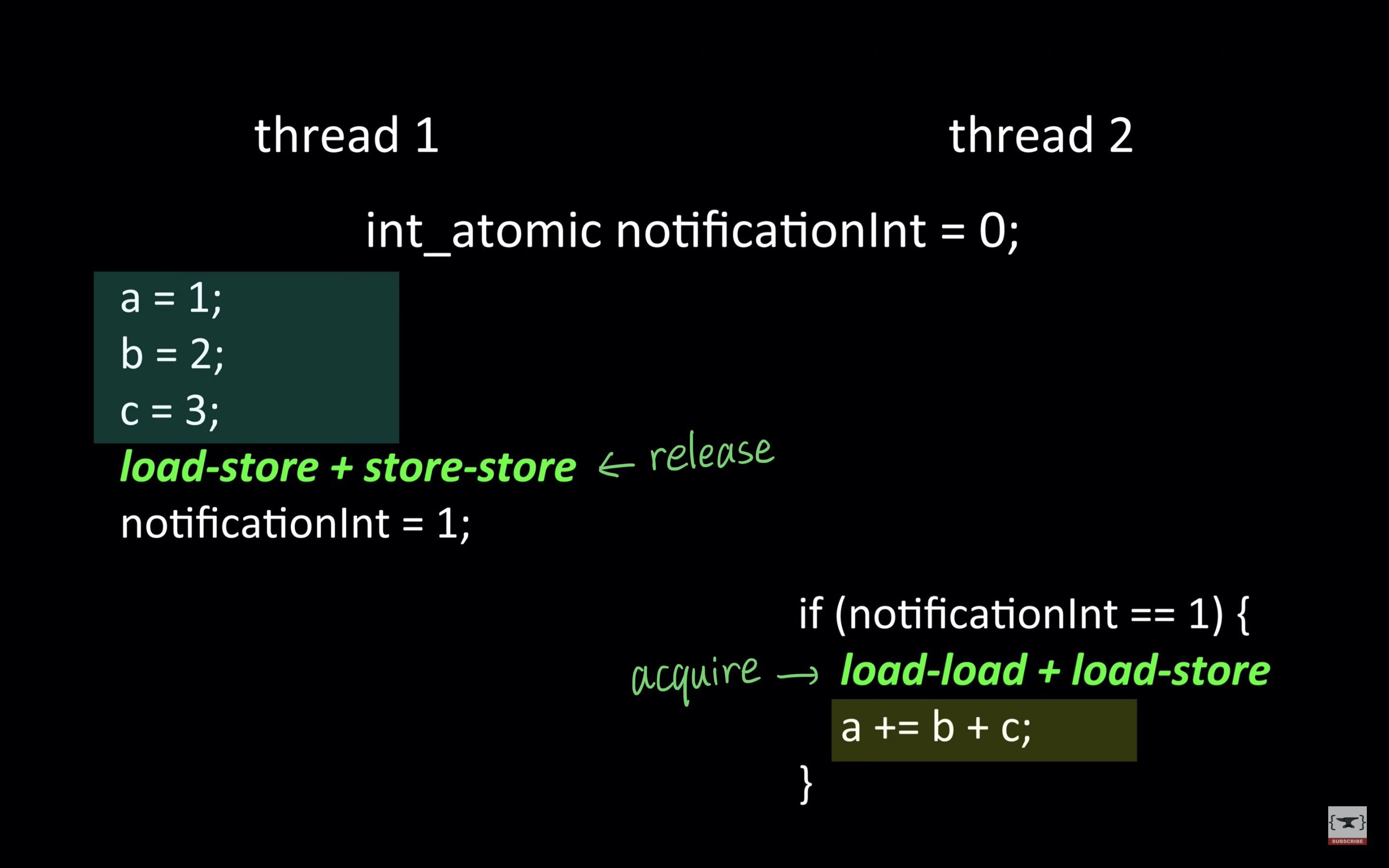
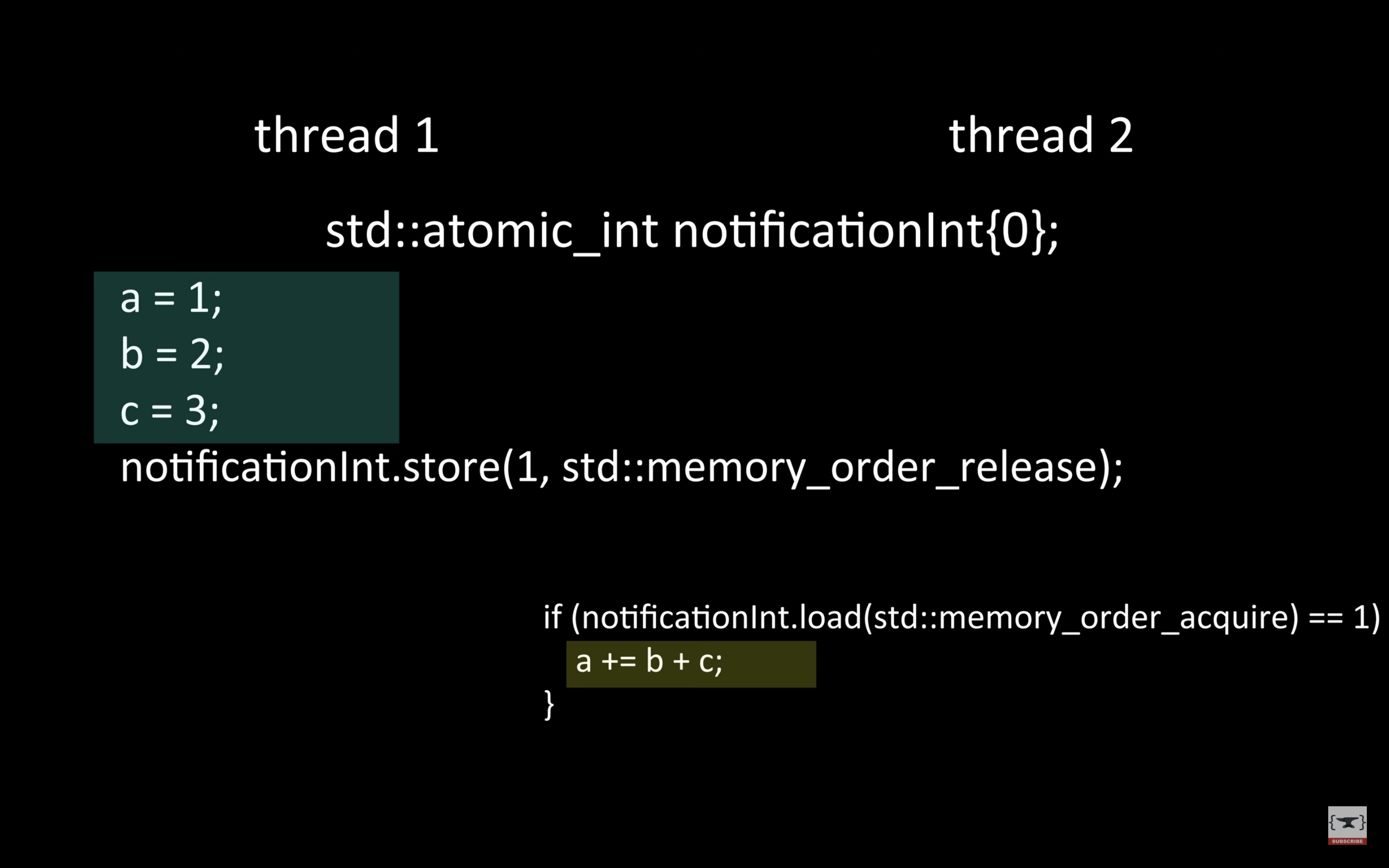