Unity Note - Project10 Wave
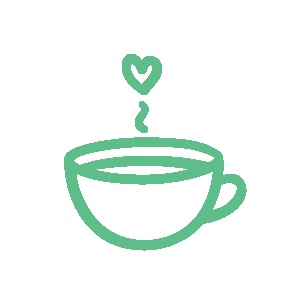
尝试做个波浪动画,最后效果:
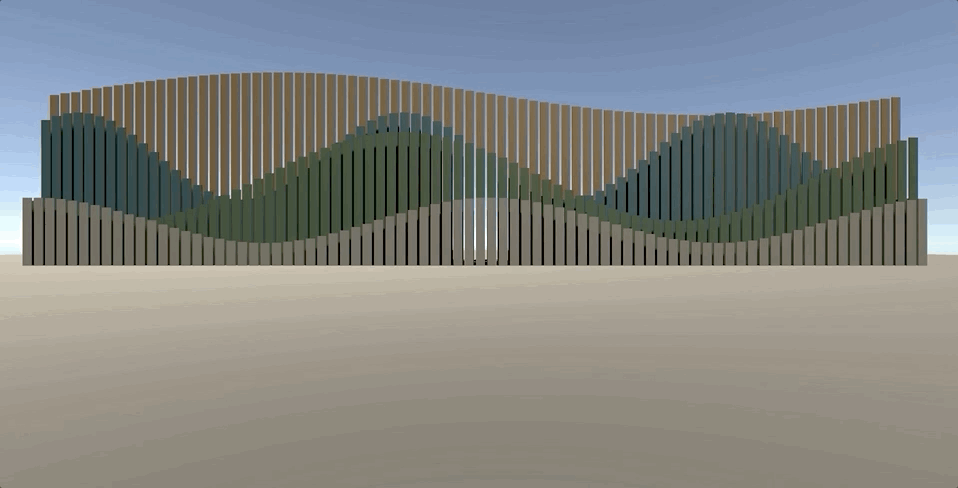
strip做成Prefab
1 | using System.Collections; |
加一个controller
1 | using System.Collections; |
奇怪的一点,前几个条的起始时间总是有点问题,暂时找不出来为什么,先把所有起始时间往后挪一点 MARK
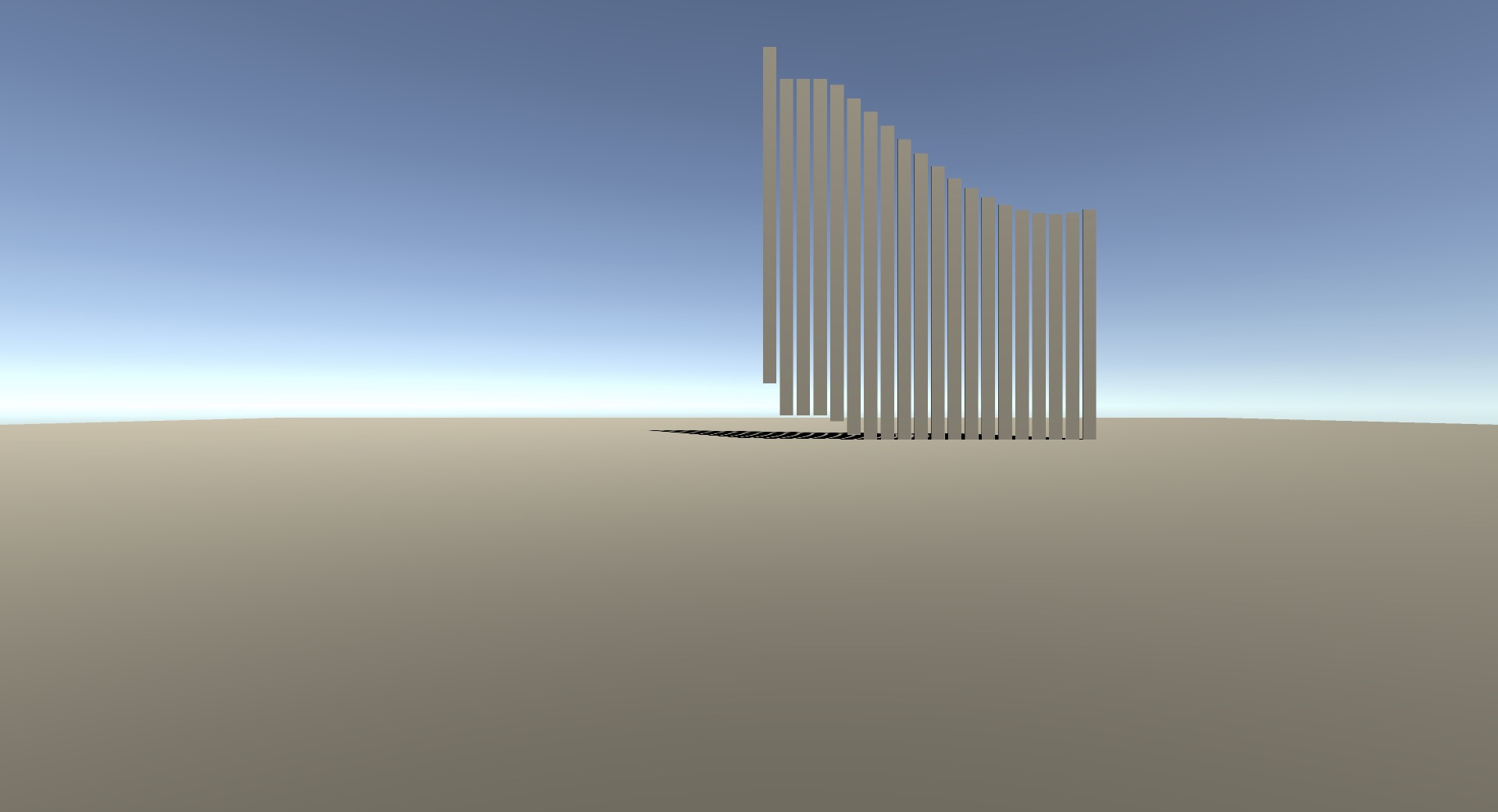
这样就没问题了
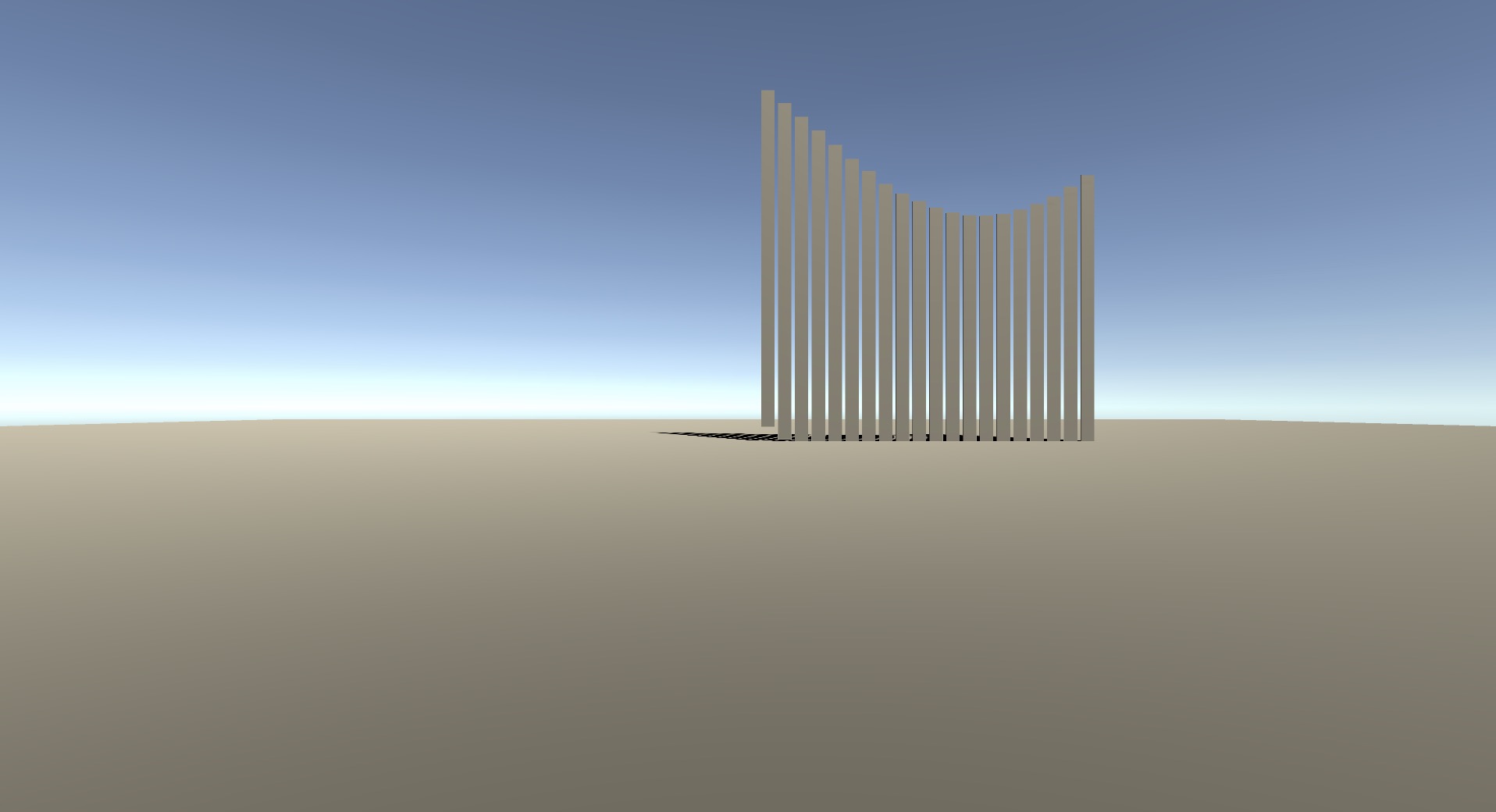
关于WaitForSeconds()
1 | private void executeWait() |
现在这个波浪效果一个问题是DOTWeen消耗太大

也许还是直接用波浪形物体平移?
一个很喜欢的chrome插件几枝是用noise做的波浪,试一下Mathf.PerlinNoise()
参考:
https://blog.csdn.net/FumikiSAMA/article/details/80212432
https://docs.unity3d.com/ScriptReference/Mathf.PerlinNoise.html
网格拓扑绘制图形
试着画了一个四面体
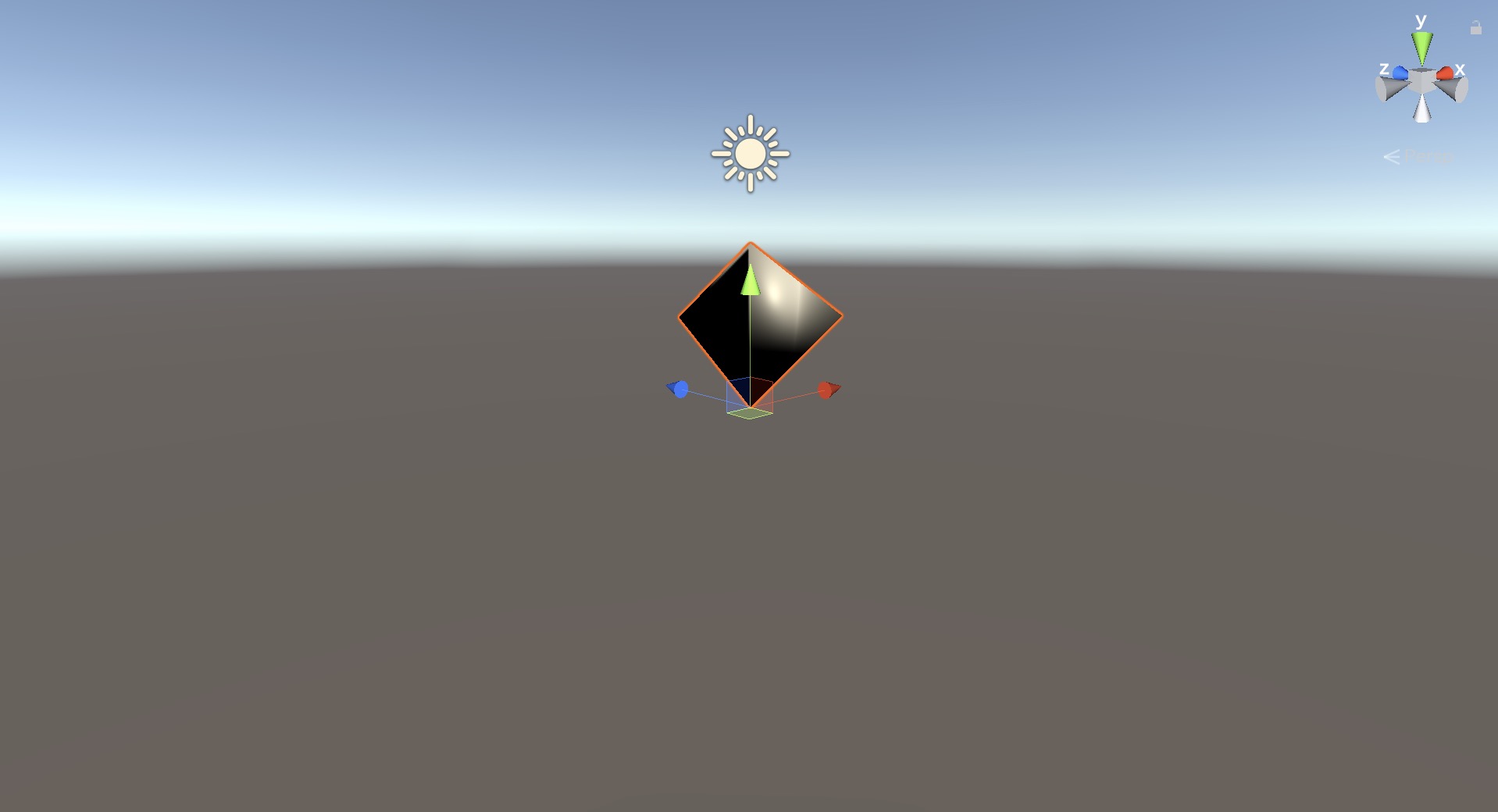
1 | Vector3[] vertices = new Vector3[4]; |
下次用这个画波浪试试